If you’re venturing into the realm of blockchain technology and modern web development, combining Next.js and Solidity can be a game-changer. This dynamic duo allows you to create powerful decentralized applications (DApps) with seamless user experiences. In this article, we'll explore how to integrate Next.js with Solidity, providing practical examples and best practices to help you get started.
Table of Contents
Introduction to Next.js and Solidity
What is Next.js?
Next.js is a popular React framework known for its server-side rendering, static site generation, and API routes. It simplifies the development of React applications by offering built-in features like automatic code splitting, optimized performance, and easy deployment.
What is Solidity?
Solidity is a statically-typed programming language used for writing smart contracts on the Ethereum blockchain. Smart contracts are self-executing contracts with the terms of the agreement directly written into code, enabling secure and decentralized transactions without intermediaries.
Why Combine Next.js and Solidity?
By combining Next.js and Solidity, you can build decentralized applications with a modern frontend framework while leveraging blockchain technology for secure, transparent, and tamper-proof backend logic. This combination allows for creating user-friendly interfaces that interact seamlessly with smart contracts.
Setting Up Your Development Environment
Prerequisites
To get started, ensure you have the following installed:
- Node.js and npm: Node.js is a JavaScript runtime, and npm is its package manager.
- Truffle: A development framework for Ethereum.
- Ganache: A personal blockchain for Ethereum development.
- MetaMask: A browser extension for interacting with the Ethereum network.
Installing Node.js and npm
Download and install Node.js and npm from the official Node.js website.
Installing Truffle and Ganache
Install Truffle and Ganache using npm:
1 2 |
npm install -g truffle npm install -g ganache-cli |
Installing MetaMask
Install MetaMask from the official MetaMask website and create an account.
Writing and Deploying a Simple Solidity Smart Contract
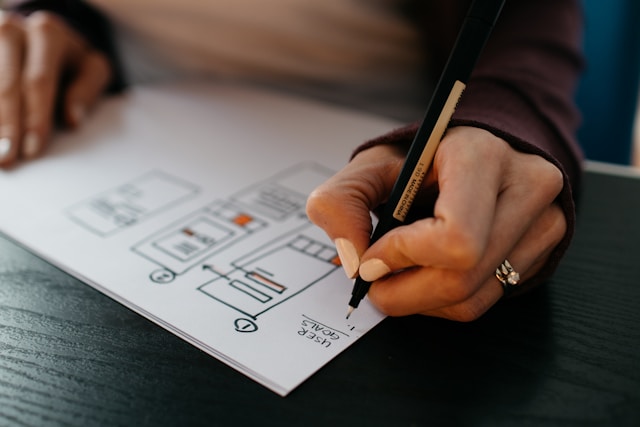
Creating a New Truffle Project
Initialize a new Truffle project:
1 2 3 |
mkdir MyDApp cd MyDApp truffle init |
Writing a Simple Smart Contract
Create a new Solidity file in the contracts
directory called SimpleStorage.sol
:
1 2 3 4 5 6 7 8 9 10 11 12 13 |
pragma solidity ^0.8.0; contract SimpleStorage { uint256 public storedData; function set(uint256 x) public { storedData = x; } function get() public view returns (uint256) { return storedData; } } |
This contract defines a state variable storedData
and two functions: set
to update the value and get
to retrieve it.
Compiling and Migrating the Smart Contract
Compile the smart contract:
1 |
truffle compile |
Create a migration script in the migrations
directory called 2_deploy_contracts.js
:
1 2 3 4 5 |
const SimpleStorage = artifacts.require("SimpleStorage"); module.exports = function (deployer) { deployer.deploy(SimpleStorage); }; |
Deploy the smart contract to Ganache:
1 2 |
ganache-cli truffle migrate |
Creating a Next.js Frontend
Setting Up a New Next.js Project
Create a new Next.js project using Create Next App:
1 2 |
npx create-next-app my-dapp cd my-dapp |
Installing Web3.js
Web3.js is a library that allows you to interact with the Ethereum blockchain from your frontend application. Install Web3.js:
1 |
npm install web3 |
Connecting to the Blockchain
Create a new file called web3.js
in the lib
directory to set up Web3:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 |
import Web3 from "web3"; const getWeb3 = () => new Promise((resolve, reject) => { window.addEventListener("load", async () => { if (window.ethereum) { const web3 = new Web3(window.ethereum); try { await window.ethereum.request({ method: "eth_requestAccounts" }); resolve(web3); } catch (error) { reject(error); } } else if (window.web3) { resolve(window.web3); } else { reject(new Error("Must install MetaMask")); } }); }); export default getWeb3; |
Interacting with the Smart Contract
Create a new file called SimpleStorage.js
in the lib
directory to interact with the smart contract:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 |
import getWeb3 from "./web3"; import SimpleStorageContract from "./SimpleStorage.json"; const getContract = async () => { const web3 = await getWeb3(); const networkId = await web3.eth.net.getId(); const deployedNetwork = SimpleStorageContract.networks[networkId]; const contract = new web3.eth.Contract( SimpleStorageContract.abi, deployedNetwork && deployedNetwork.address ); return contract; }; export default getContract; |
Creating the React Components
Create a simple interface to interact with the smart contract. In pages/index.js
, add the following code:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 |
import React, { useState, useEffect } from "react"; import getWeb3 from "../lib/web3"; import getContract from "../lib/SimpleStorage"; const Home = () => { const [storageValue, setStorageValue] = useState(0); const [inputValue, setInputValue] = useState(""); const [web3, setWeb3] = useState(null); const [accounts, setAccounts] = useState(null); const [contract, setContract] = useState(null); useEffect(() => { const init = async () => { const web3 = await getWeb3(); const accounts = await web3.eth.getAccounts(); const contract = await getContract(); setWeb3(web3); setAccounts(accounts); setContract(contract); const response = await contract.methods.get().call(); setStorageValue(response); }; init(); }, []); const handleInputChange = (event) => { setInputValue(event.target.value); }; const handleSubmit = async () => { await contract.methods.set(inputValue).send({ from: accounts[0] }); const response = await contract.methods.get().call(); setStorageValue(response); }; return ( <div> <h1>Simple Storage DApp</h1> <p>Stored Value: {storageValue}</p> <input type="number" value={inputValue} onChange={handleInputChange} /> <button onClick={handleSubmit}>Set Value</button> </div> ); }; export default Home; |
Running the Next.js Application
Start your Next.js application:
1 |
npm run dev |
Open your browser and navigate to http://localhost:3000
to see your DApp in action. You should be able to interact with the smart contract by setting and getting the stored value.
Best Practices for Developing with Next.js and Solidity
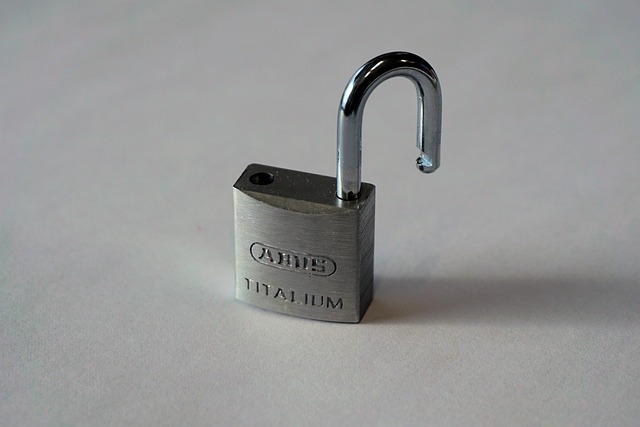
Efficient State Management
Efficiently manage the state of your application to ensure optimal performance. Utilize hooks like useState
and useEffect
to manage component states and side effects.
Error Handling
Implement comprehensive error handling to improve the user experience. Display informative messages to users when transactions fail or when they need to connect their wallet.
Gas Optimization
Optimize your smart contracts to reduce gas costs. Avoid unnecessary storage operations and batch multiple operations into a single transaction when possible.
Security Best Practices
Security is paramount in smart contract development. Follow best practices such as using OpenZeppelin's library for secure contract patterns, avoiding reentrancy attacks, and thoroughly testing your contracts.
Testing Your Contracts
Thoroughly test your smart contracts using frameworks like Truffle or Hardhat. Write unit tests to ensure your contracts behave as expected under different scenarios.
Example of a test file using Truffle:
1 2 3 4 5 6 7 8 9 10 |
const SimpleStorage = artifacts.require("SimpleStorage"); contract("SimpleStorage", (accounts) => { it("should store the value 42", async () => { const instance = await SimpleStorage.deployed(); await instance.set(42, { from: accounts[0] }); const storedData = await instance.get.call(); assert.equal(storedData, 42, "The value 42 was not stored."); }); }); |
Conclusion
Combining Next.js and Solidity allows you to build powerful decentralized applications with a modern frontend and a secure, transparent backend. This article has provided a comprehensive guide to setting up your development environment, writing and deploying smart contracts, and creating a user-friendly interface with Next.js. By following best practices and optimizing your code, you can create efficient and secure DApps that leverage the full potential of blockchain technology.