Ethereum has revolutionized the world of decentralized applications with its robust smart contract capabilities. Solidity, the programming language for Ethereum, has become an essential tool for anyone looking to dive into blockchain development. This guide will introduce the basics of Solidity, providing a comprehensive overview suitable for digital nomads, programmers, and data scientists.
Table of Contents
Introduction to Solidity
What is Solidity?
Solidity is a statically-typed programming language designed for developing smart contracts that run on the Ethereum Virtual Machine (EVM). It is influenced by JavaScript, Python, and C++, making it relatively easy for developers familiar with these languages to learn.
Why Learn Solidity?
For digital nomads, programmers, and data scientists, learning Solidity opens up opportunities to develop decentralized applications (dApps), engage in decentralized finance (DeFi), and contribute to blockchain projects. The demand for Solidity developers is high, and mastering this language can significantly enhance your career prospects.
Setting Up the Development Environment
Before we dive into coding, let's set up our development environment.
Install Node.js and npm:
Node.js and npm (Node Package Manager) are required for most Ethereum development tools. Install them from the official Node.js website.
Install Truffle and Ganache:
Truffle is a popular development framework for Ethereum, and Ganache is a personal blockchain for Ethereum development.
1 2 |
npm install -g truffle npm install -g ganache-cli |
Install MetaMask:
MetaMask is a browser extension that allows you to interact with the Ethereum blockchain. Install it from the official MetaMask website and create an account.
Writing Your First Smart Contract
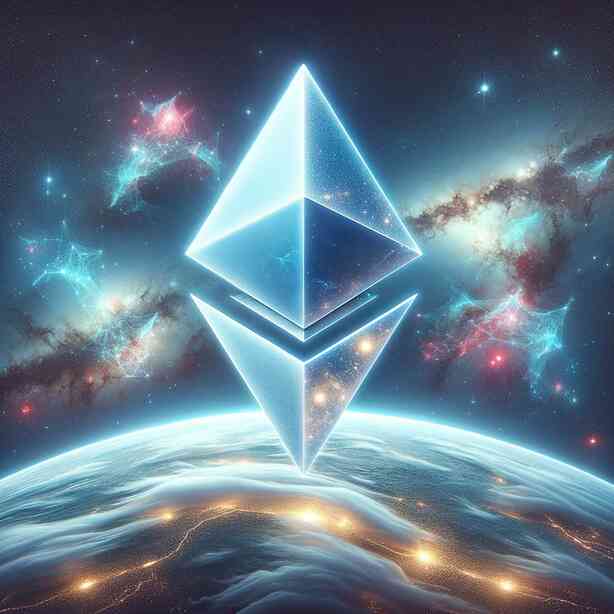
Smart Contract Basics
A smart contract is a collection of code and data that resides at a specific address on the Ethereum blockchain. Solidity is used to write these contracts.
Hello World Contract
Here’s a simple "Hello World" contract:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 |
// SPDX-License-Identifier: MIT pragma solidity ^0.8.0; contract HelloWorld { string public greeting; constructor() { greeting = "Hello, World!"; } function setGreeting(string memory _greeting) public { greeting = _greeting; } function getGreeting() public view returns (string memory) { return greeting; } } |
Explanation
- SPDX License Identifier: Specifies the license for the code.
- Pragma: Indicates the version of Solidity.
- Contract: Defines a new contract.
- State Variable:
greeting
stores a string. - Constructor: Initializes the state variable.
- Functions:
setGreeting
changes the greeting, andgetGreeting
returns the current greeting.
Data Types in Solidity
Value Types
Solidity supports various value types, which are basic types that hold single values. These include:
- Boolean:
bool
- Represents true or false. - Integer:
int
anduint
- Signed and unsigned integers of various sizes. - Address:
address
- Represents a 20-byte Ethereum address. - Bytes:
bytes1
tobytes32
- Fixed-size byte arrays.
Here's a table summarizing value types:
Type | Description | Example |
---|---|---|
bool |
Boolean value | bool isReady; |
int |
Signed integer | int count; |
uint |
Unsigned integer | uint age; |
address |
Ethereum address | address wallet; |
bytes |
Fixed-size byte array | bytes32 data; |
Reference Types
Reference types include more complex types like arrays, structs, and mappings.
Arrays
Arrays in Solidity can be of fixed or dynamic size.
- Fixed-size Array:
1 |
uint[5] public fixedArray; |
- Dynamic Array:
1 |
uint[] public dynamicArray; |
Structs
Structs are custom data types that group together related variables.
1 2 3 4 5 6 |
struct Person { string name; uint age; } Person public person; |
Mappings
Mappings are key-value stores where keys are mapped to values. They are particularly useful for associative arrays.
1 |
mapping(address => uint) public balances; |
Here's a table summarizing reference types:
Type | Description | Example |
---|---|---|
Array |
Fixed or dynamic size array | uint[] numbers; |
Struct |
Custom data structure | struct Person |
Mapping |
Key-value store | mapping(address => uint) balances; |
Keywords and Operators
Solidity includes various keywords and operators essential for programming.
Keywords
Here are some common keywords:
Keyword | Description |
---|---|
contract |
Defines a new contract |
function |
Defines a function |
return |
Specifies the return value of a function |
if , else |
Conditional statements |
for , while |
Loop constructs |
public , private , internal , external |
Function visibility |
Operators
Solidity supports standard arithmetic, logical, and comparison operators.
Operator | Description | Example |
---|---|---|
+ |
Addition | a + b |
- |
Subtraction | a - b |
* |
Multiplication | a * b |
/ |
Division | a / b |
== |
Equality | a == b |
!= |
Inequality | a != b |
&& |
Logical AND | a && b |
|| |
Logical OR | a || b |
Control Structures
Solidity supports typical control structures like if
, else
, for
, while
, and do-while
.
1 2 3 4 5 6 7 |
function checkEven(uint _number) public pure returns (bool) { if (_number % 2 == 0) { return true; } else { return false; } } |
Functions
Functions are executable units of code. Solidity supports various types of functions:
- Public Functions: Accessible from anywhere.
- Private Functions: Accessible only within the contract.
- Internal Functions: Accessible within the contract and derived contracts.
- External Functions: Accessible only from other contracts or transactions.
1 2 3 4 |
function publicFunction() public {} function privateFunction() private {} function internalFunction() internal {} function externalFunction() external {} |
Deploying Smart Contracts
Using Truffle
Truffle simplifies the deployment process.
Initialize a Truffle Project:
1 2 3 |
mkdir MyProject cd MyProject truffle init |
Compile the Contract:
Place your contract in the contracts
directory and compile it:
1 |
truffle compile |
Deploy the Contract:
Create a migration script in the migrations
directory:
1 2 3 4 5 |
const HelloWorld = artifacts.require("HelloWorld"); module.exports = function (deployer) { deployer.deploy(HelloWorld); }; |
Deploy the contract:
1 |
truffle migrate |
Using Ganache
Ganache provides a local blockchain for testing.
Start Ganache:
1 |
ganache-cli |
Connect Truffle to Ganache:
Configure Truffle to use Ganache in truffle-config.js
:
1 2 3 4 5 6 7 8 9 |
module.exports = { networks: { development: { host: "127.0.0.1", port: 8545, network_id: "*", // Match any network id }, }, }; |
Deploy to Ganache:
Deploy your contract using Truffle:
1 |
truffle migrate --network development |
Interacting with Smart Contracts
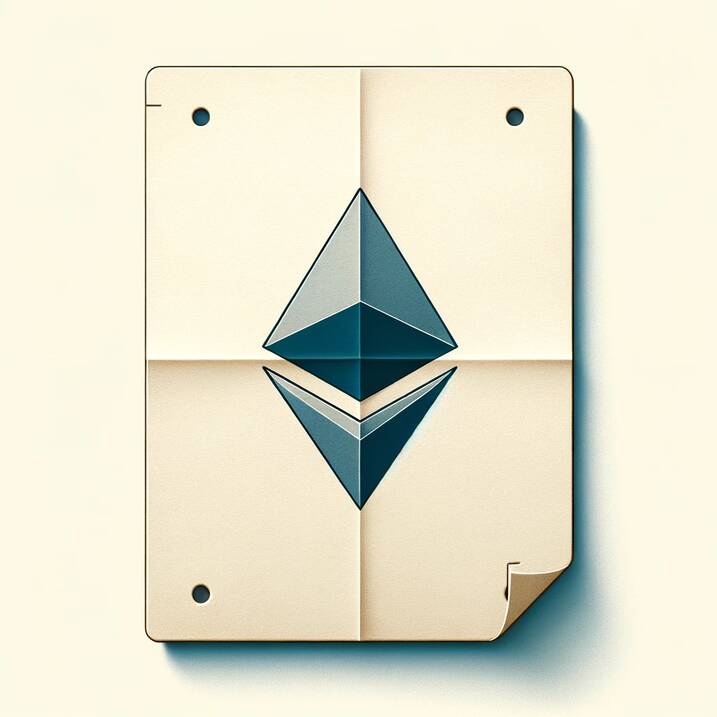
Using Web3.js and JavaScript
Web3.js is a library that allows you to interact with the Ethereum blockchain.
Install Web3.js:
1 |
npm install web3 |
Interact with the Contract:
1 2 3 4 5 6 7 8 9 10 11 12 13 |
const Web3 = require("web3"); const web3 = new Web3("http://localhost:8545"); const abi = [/* ABI from compiled contract */]; const address = "/* Deployed contract address */"; const contract = new web3.eth.Contract(abi, address); async function getGreeting() { const greeting = await contract.methods.getGreeting().call(); console.log(greeting); } getGreeting(); |
Advanced Topics
Inheritance
Solidity supports inheritance, allowing contracts to inherit properties and methods from other contracts.
1 2 3 4 5 6 7 8 9 10 11 |
contract Parent { function parentFunction() public pure returns (string memory) { return "Parent"; } } contract Child is Parent { function childFunction() public pure returns (string memory) { return "Child"; } } |
Modifiers
Modifiers are used to change the behavior of functions.
1 2 3 4 5 6 7 8 |
modifier onlyOwner() { require(msg.sender == owner, "Not the owner"); _; } function restrictedFunction() public onlyOwner { // Function logic } |
Events
Events allow logging on the Ethereum blockchain.
1 2 3 4 5 6 7 8 |
event GreetingChanged(string newGreeting); function setGreeting(string memory _greeting) public { greeting = _greeting; emit GreetingChanged(_greeting); } |
Libraries
Libraries are reusable code that can be deployed once and linked to other contracts.
1 2 3 4 5 6 7 8 9 10 11 12 13 |
library Math { function add(uint a, uint b) internal pure returns (uint) { return a + b; } } contract Calculator { using Math for uint; function calculate(uint a, uint b) public pure returns (uint) { return a.add(b); } } |
Conclusion
Solidity is a powerful language that enables the development of smart contracts on the Ethereum blockchain. This guide has introduced the basics of Solidity, including data types, control structures, functions, and contract deployment. For digital nomads, programmers, and data scientists, mastering Solidity opens up new opportunities in the rapidly growing field of blockchain technology. Keep experimenting with more complex contracts and dive deeper into the advanced features to become proficient in Solidity. Happy coding!