Developing decentralized applications (DApps) has become increasingly popular, especially among digital nomads, programmers, and data scientists. Combining the power of Solidity for smart contract development and React.js for building user interfaces provides a robust framework for creating innovative DApps. This guide will walk you through the process of building a DApp using Solidity and React.js, with practical examples and sample code to help you get started.
Table of Contents
Introduction to DApp Development
What is a DApp?
A decentralized application (DApp) is a software application that runs on a decentralized network, such as Ethereum and Polygon, rather than being hosted on centralized servers. DApps leverage blockchain technology to offer greater security, transparency, and user control.
Why Use Solidity and React.js?
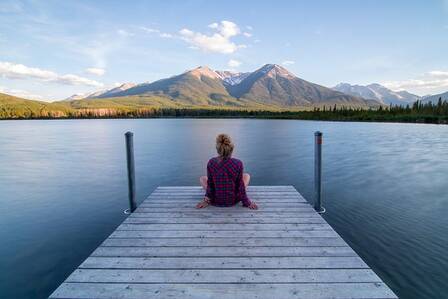
- Solidity: Solidity is the primary language for writing smart contracts on the Ethereum blockchain. It allows you to create secure and reliable contracts that can execute automatically.
- React.js: React.js is a popular JavaScript library for building user interfaces. It provides a flexible and efficient way to create dynamic and interactive front-end applications.
Combining Solidity and React.js enables developers to build comprehensive DApps with a secure backend and a responsive front-end.
Setting Up Your Development Environment
Prerequisites
Before you begin, ensure you have the following installed:
- Node.js and npm: Node.js is a JavaScript runtime, and npm is its package manager.
- Truffle: A development framework for Ethereum.
- Ganache: A personal blockchain for Ethereum development.
- MetaMask: A browser extension for interacting with the Ethereum network.
Installing Node.js and npm
Download and install Node.js and npm from the official Node.js website.
Installing Truffle and Ganache
Install Truffle and Ganache using npm:
1 2 |
npm install -g truffle npm install -g ganache-cli |
Installing MetaMask
Install MetaMask from the official MetaMask website and create an account.
Writing Smart Contracts with Solidity
Creating a New Truffle Project
Create a new Truffle project and initialize it:
1 2 3 |
mkdir MyDApp cd MyDApp truffle init |
Writing a Simple Smart Contract
Create a new Solidity file in the contracts
directory called SimpleStorage.sol
:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 |
// SPDX-License-Identifier: MIT pragma solidity ^0.8.0; contract SimpleStorage { uint public storedData; function set(uint x) public { storedData = x; } function get() public view returns (uint) { return storedData; } } |
Compiling and Migrating the Smart Contract
Compile the smart contract:
1 |
truffle compile |
Create a migration script in the migrations
directory called 2_deploy_contracts.js
:
1 2 3 4 5 |
const SimpleStorage = artifacts.require("SimpleStorage"); module.exports = function(deployer) { deployer.deploy(SimpleStorage); }; |
Deploy the smart contract to Ganache:
1 2 |
ganache-cli truffle migrate |
Developing the Front-End with React.js
Creating a New React.js Project
Create a new React.js project using Create React App:
1 2 |
npx create-react-app my-dapp cd my-dapp |
Installing Web3.js
Web3.js is a library that allows you to interact with the Ethereum blockchain from your front-end application. Install Web3.js:
1 |
npm install web3 |
Connecting to the Blockchain
Create a new file called web3.js
in the src
directory to set up Web3:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 |
import Web3 from "web3"; const getWeb3 = () => new Promise((resolve, reject) => { window.addEventListener("load", async () => { if (window.ethereum) { const web3 = new Web3(window.ethereum); try { await window.ethereum.request({ method: "eth_requestAccounts" }); resolve(web3); } catch (error) { reject(error); } } else if (window.web3) { resolve(window.web3); } else { reject(new Error("Must install MetaMask")); } }); }); export default getWeb3; |
Interacting with the Smart Contract
Create a new file called SimpleStorage.js
in the src
directory to interact with the smart contract:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 |
import getWeb3 from "./web3"; import SimpleStorageContract from "./contracts/SimpleStorage.json"; const getContract = async () => { const web3 = await getWeb3(); const networkId = await web3.eth.net.getId(); const deployedNetwork = SimpleStorageContract.networks[networkId]; const contract = new web3.eth.Contract( SimpleStorageContract.abi, deployedNetwork && deployedNetwork.address ); return contract; }; export default getContract; |
Creating the React Components
Create a simple interface to interact with the smart contract. In src/App.js
, add the following code:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 |
import React, { useState, useEffect } from "react"; import getWeb3 from "./web3"; import getContract from "./SimpleStorage"; const App = () => { const [storageValue, setStorageValue] = useState(0); const [inputValue, setInputValue] = useState(""); const [web3, setWeb3] = useState(null); const [accounts, setAccounts] = useState(null); const [contract, setContract] = useState(null); useEffect(() => { const init = async () => { const web3 = await getWeb3(); const accounts = await web3.eth.getAccounts(); const contract = await getContract(); setWeb3(web3); setAccounts(accounts); setContract(contract); const response = await contract.methods.get().call(); setStorageValue(response); }; init(); }, []); const handleInputChange = (event) => { setInputValue(event.target.value); }; const handleSubmit = async () => { await contract.methods.set(inputValue).send({ from: accounts[0] }); const response = await contract.methods.get().call(); setStorageValue(response); }; return ( <div> <h1>Simple Storage DApp</h1> <p>Stored Value: {storageValue}</p> <input type="number" value={inputValue} onChange={handleInputChange} /> <button onClick={handleSubmit}>Set Value</button> </div> ); }; export default App; |
Running the React.js Application
Start your React.js application:
1 |
npm start |
Open your browser and navigate to http://localhost:3000
to see your DApp in action. You should be able to interact with the smart contract by setting and getting the stored value.
Advanced Topics
Handling Events in Solidity and React.js
Solidity contracts can emit events that can be listened to by your React.js application. Modify the SimpleStorage.sol
contract to emit an event when the value is set:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 |
// SPDX-License-Identifier: MIT pragma solidity ^0.8.0; contract SimpleStorage { uint public storedData; event ValueChanged(uint newValue); function set(uint x) public { storedData = x; emit ValueChanged(x); } function get() public view returns (uint) { return storedData; } } |
Update SimpleStorage.js
to listen for the ValueChanged
event:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 |
const listenForEvents = (contract) => { contract.events.ValueChanged({}, (error, event) => { if (!error) { console.log("Event received:", event.returnValues); } }); }; const init = async () => { const web3 = await getWeb3(); const accounts = await web3.eth.getAccounts(); const contract = await getContract(); setWeb3(web3); setAccounts(accounts); setContract(contract); const response = await contract.methods.get().call(); setStorageValue(response); listenForEvents(contract); }; |
Integrating Additional Features
Consider adding more features to your DApp, such as:
- User Authentication: Use MetaMask to authenticate users.
- Data Visualization: Display stored data using charts and graphs.
- Smart Contract Interaction: Allow users to interact with multiple smart contracts.
Deployment to a Testnet
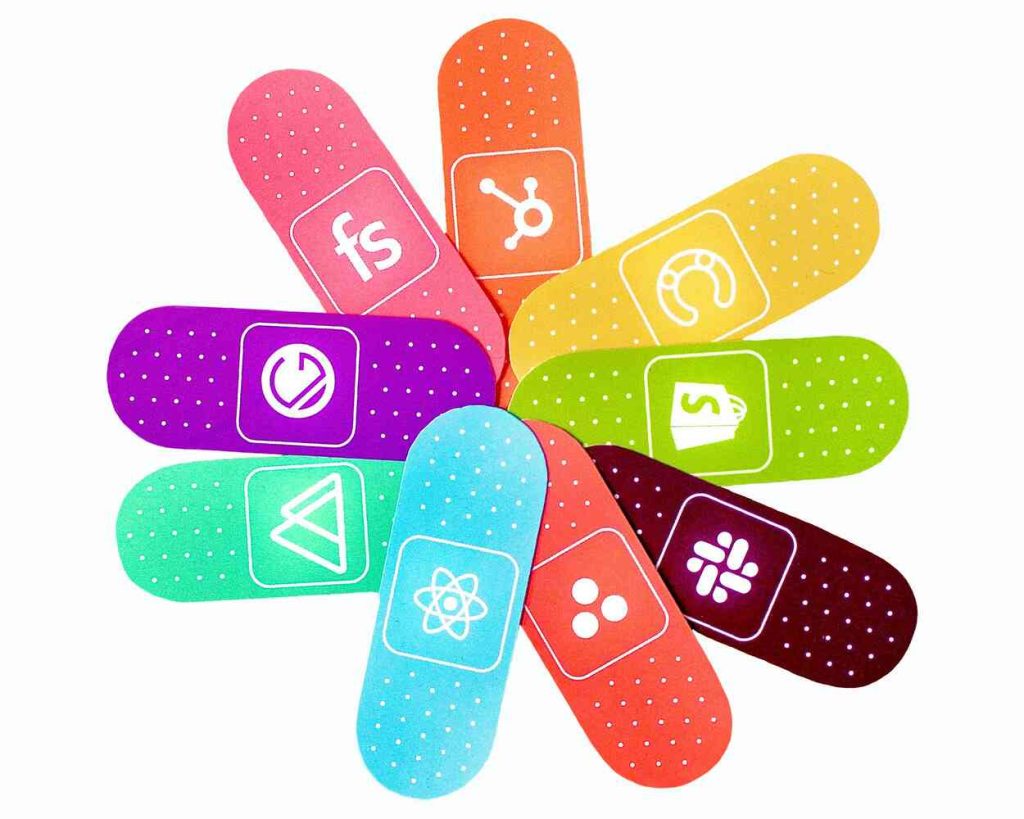
Once your DApp is ready, you can deploy it to a testnet like Ropsten or Rinkeby. Update your Truffle configuration to use an Infura endpoint:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 |
const HDWalletProvider = require("@truffle/hdwallet-provider"); const infuraKey = "YOUR_INFURA_PROJECT_ID"; const mnemonic = "YOUR_METAMASK_MNEMONIC"; module.exports = { networks: { ropsten: { provider: () => new HDWalletProvider(mnemonic, `https://ropsten.infura.io/v3/${infuraKey}`), network_id: 3, }, rinkeby: { provider: () => new HDWalletProvider(mnemonic, `https://rinkeby.infura.io/v3/${infuraKey}`), network_id: 4, }, }, compilers: { solc: { version: "0.8.0", }, }, }; |
Deploy your contract to the desired testnet:
1 |
truffle migrate --network ropsten |
Conclusion
Building a DApp using Solidity and React.js combines the strengths of blockchain technology and modern web development to create secure, decentralized applications. This guide has provided a comprehensive overview of setting up your development environment, writing and deploying smart contracts, and building a React.js front end. By leveraging these technologies, digital nomads, programmers, and data scientists can develop innovative solutions that harness the power of decentralization.