Welcome to the world of Ethereum and smart contract development with Solidity! If you're diving into blockchain technology and decentralized applications, understanding how to write efficient and secure smart contracts is essential. This article will explore key concepts such as Wei, gas price estimation, bytecode, and the Solidity compiler. By the end, you'll have a solid grasp of these elements and how they contribute to building robust Ethereum applications.
Table of Contents
Introduction to Solidity and Ethereum
Solidity is the statically-typed programming language specifically designed for writing smart contracts on the Ethereum blockchain. Ethereum, a decentralized platform, allows developers to build and deploy smart contracts, which are self-executing contracts with the terms of the agreement directly written into code. These contracts automatically enforce and execute agreements without the need for intermediaries.
Understanding Wei and Gas
Wei: The Smallest Unit of Ether
Ether (ETH) is the native cryptocurrency of the Ethereum network. Similar to how cents are to dollars, Wei is the smallest unit of Ether. One Ether is equal to (10^{18}) Wei. Using Wei in smart contracts is crucial because it ensures precision in financial calculations, avoiding issues with floating-point arithmetic.
Gas: The Fuel for Transactions
Gas is the unit that measures the amount of computational effort required to execute operations in the Ethereum network. Each operation performed by a smart contract consumes a certain amount of gas. The total gas used in a transaction determines the transaction fee, which is paid in Ether.
Gas fees are calculated as follows:
[ \text{Gas Fee} = \text{Gas Used} \times \text{Gas Price} ]
Gas Price Estimation
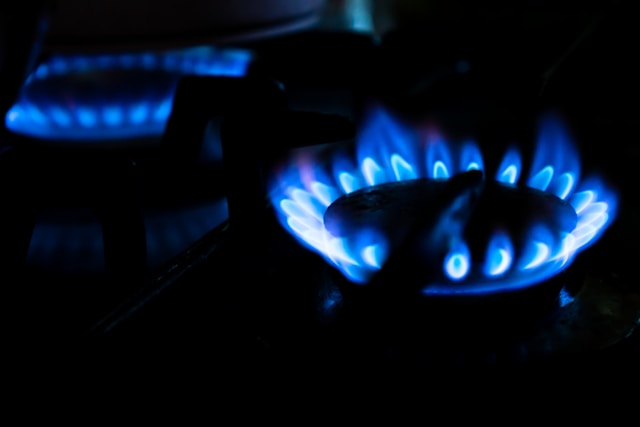
The gas price is the amount of Ether you are willing to pay per unit of gas. It is measured in Gwei, where (1 \text{ Gwei} = 10^9 \text{ Wei}). Higher gas prices incentivize miners to prioritize your transaction.
Estimating gas prices can be done using various Ethereum tools and libraries. For example, you can use the Web3.js library to fetch current gas prices:
1 2 3 4 5 6 7 8 9 |
const Web3 = require('web3'); const web3 = new Web3('https://mainnet.infura.io/v3/YOUR_INFURA_PROJECT_ID'); async function getGasPrice() { const gasPrice = await web3.eth.getGasPrice(); console.log('Current Gas Price:', gasPrice); } getGasPrice(); |
This script connects to the Ethereum mainnet via Infura and logs the current gas price.
Writing and Compiling Smart Contracts with Solidity
Setting Up the Solidity Compiler
To compile Solidity code, you need the Solidity compiler (solc
). You can install solc
on your machine using npm:
1 |
npm install -g solc |
Alternatively, if you prefer using Remix, an online Solidity IDE, it includes an integrated compiler and provides an easy way to write, compile, and deploy contracts.
Writing a Simple Smart Contract
Let's start with a simple smart contract that stores and retrieves a value.
1 2 3 4 5 6 7 8 9 10 11 12 13 |
pragma solidity ^0.8.0; contract SimpleStorage { uint256 public storedData; function set(uint256 x) public { storedData = x; } function get() public view returns (uint256) { return storedData; } } |
This contract defines a state variable storedData
and two functions: set
to update the value and get
to retrieve it.
Compiling the Smart Contract
To compile the contract using solc
, save the code to a file named SimpleStorage.sol
and run:
1 |
solc --bin --abi --optimize -o build SimpleStorage.sol |
This command generates the contract's bytecode and ABI (Application Binary Interface) and saves them in the build
directory. The bytecode is the compiled code that gets deployed to the Ethereum network, while the ABI is a JSON representation of the contract's interface, allowing you to interact with it programmatically.
Understanding Bytecode
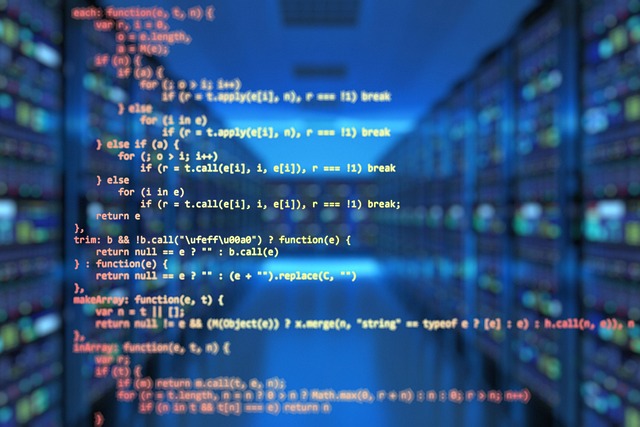
Bytecode is the low-level representation of your smart contract that is executed by the Ethereum Virtual Machine (EVM). When you deploy a contract, its bytecode is stored on the blockchain, and the EVM interprets this bytecode to execute the contract's functions.
Deploying and Interacting with Bytecode
You can deploy the compiled bytecode using Web3.js. First, ensure you have an Ethereum account with some ETH for gas fees.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 |
const Web3 = require('web3'); const web3 = new Web3('https://mainnet.infura.io/v3/YOUR_INFURA_PROJECT_ID'); const bytecode = 'YOUR_CONTRACT_BYTECODE'; const abi = [/* YOUR_CONTRACT_ABI */]; const deployContract = async () => { const accounts = await web3.eth.getAccounts(); const myContract = new web3.eth.Contract(abi); myContract.deploy({ data: bytecode, }) .send({ from: accounts[0], gas: 1500000, gasPrice: '30000000000' // 30 Gwei }) .then((newContractInstance) => { console.log('Contract deployed at:', newContractInstance.options.address); }); }; deployContract(); |
This script deploys the contract to the Ethereum network and logs the address where the contract is deployed.
Interacting with Deployed Contracts
After deployment, you can interact with the contract using its ABI and address. For example, calling the set
function to store a value:
1 2 3 4 5 6 7 8 9 10 11 12 13 |
const myContract = new web3.eth.Contract(abi, 'CONTRACT_ADDRESS'); const setValue = async (value) => { const accounts = await web3.eth.getAccounts(); await myContract.methods.set(value).send({ from: accounts[0], gas: 100000, gasPrice: '20000000000' // 20 Gwei }); console.log('Value set to:', value); }; setValue(42); |
Estimating Gas Usage
Gas Estimation Techniques
Estimating the gas usage of your smart contract functions is crucial to ensure you have enough Ether to execute them. Web3.js provides a method to estimate gas usage:
1 2 3 4 5 6 |
const estimateGasUsage = async () => { const gasEstimate = await myContract.methods.set(42).estimateGas(); console.log('Estimated Gas:', gasEstimate); }; estimateGasUsage(); |
This script estimates the gas required to execute the set
function and logs the result.
Optimizing Gas Usage
To optimize gas usage, consider the following best practices:
- Minimize state variable updates: Each write operation to the blockchain storage costs gas.
- Use memory instead of storage for temporary variables: Accessing memory is cheaper than accessing storage.
- Batch operations: Combining multiple operations into one can reduce gas costs.
Testing Smart Contracts
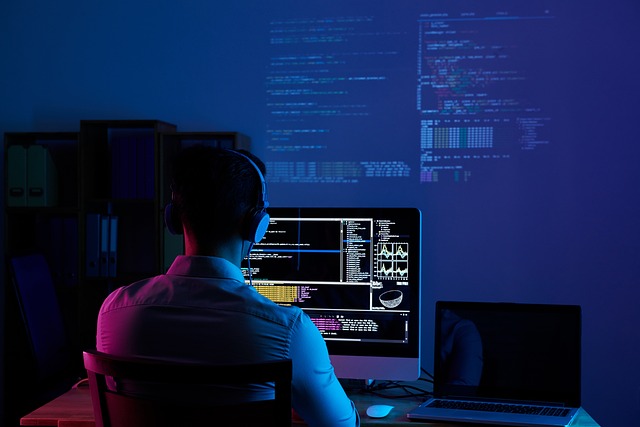
Using Truffle for Testing
Truffle is a popular development framework for Ethereum that simplifies testing and deploying smart contracts. Install Truffle using npm:
1 |
npm install -g truffle |
Initialize a new Truffle project:
1 |
truffle init |
Create a test file test/simpleStorageTest.js
:
1 2 3 4 5 6 7 8 9 10 |
const SimpleStorage = artifacts.require("SimpleStorage"); contract("SimpleStorage", (accounts) => { it("should store the value 42", async () => { const instance = await SimpleStorage.deployed(); await instance.set(42, { from: accounts[0] }); const storedData = await instance.get.call(); assert.equal(storedData, 42, "The value 42 was not stored."); }); }); |
Run the tests using Truffle:
1 |
truffle test |
This command executes the tests and provides feedback on whether the contract behaves as expected.
Writing Assertions in Tests
Using assertions in tests ensures that your smart contracts perform as intended. The assert
statement checks if a condition holds true, and if it doesn't, the test fails.
Example of an assertion:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 |
const SimpleStorage = artifacts.require("SimpleStorage"); contract("SimpleStorage", (accounts) => { it("should store the value 42", async () => { const instance = await SimpleStorage.deployed(); await instance.set(42, { from: accounts[0] }); const storedData = await instance.get.call(); assert.equal(storedData, 42, "The value 42 was not stored."); }); it("should update the value to 100", async () => { const instance = await SimpleStorage.deployed(); await instance.set(100, { from: accounts[0] }); const storedData = await instance.get.call(); assert.equal(storedData, 100, "The value 100 was not stored."); }); }); |
This test file includes assertions to check whether the values are correctly stored and retrieved from the smart contract.
Conclusion
Mastering Ethereum smart contracts with Solidity involves understanding various key concepts such as Wei, gas price estimation, bytecode, and the Solidity compiler. By diving deep into these topics, you can write efficient and secure smart contracts. This article provided a comprehensive overview of these elements with practical examples to help you get started and advance your knowledge.