In the ever-evolving world of technology, staying updated with the latest tools and frameworks is crucial for digital nomads, programmers, and data scientists. Node.js v16 and Ionic are powerful tools that can significantly enhance your development workflow, especially when building mobile applications. This comprehensive guide will walk you through the installation of Node.js v16 and Ionic on Ubuntu, providing sample codes and outputs to help you get started.
Table of Contents
Introduction
Why Node.js and Ionic?
Node.js is a powerful JavaScript runtime built on Chrome's V8 engine, known for its event-driven, non-blocking I/O model, making it ideal for building scalable network applications. With the latest v16, Node.js introduces new features and performance improvements that can significantly benefit your projects.
Ionic is a popular open-source framework for building cross-platform mobile applications using web technologies like HTML, CSS, and JavaScript. It allows you to write once and deploy everywhere, making it a favorite among digital nomads and developers targeting multiple platforms.
Combining Node.js with Ionic offers a robust environment for building, testing, and deploying mobile applications quickly and efficiently.
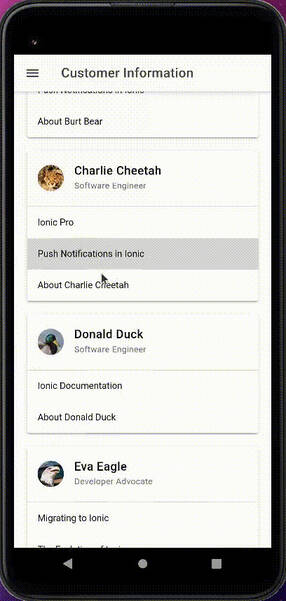
Prerequisites
Before diving into the installation, ensure you have the following:
- A machine running Ubuntu (20.04 LTS or later)
- Basic knowledge of the terminal and shell commands
- Administrative privileges to install software
Installing Node.js v16
Step 1: Update Your System
First, ensure your system is up to date by running the following commands:
1 2 |
sudo apt update sudo apt upgrade -y |
Step 2: Install Node.js v16
Node.js v16 can be installed using NodeSource’s binary distributions. Run the following commands to add the NodeSource repository and install Node.js v16:
1 2 |
curl -fsSL https://deb.nodesource.com/setup_16.x | sudo -E bash - sudo apt install -y nodejs |
Verify the installation by checking the Node.js and npm (Node Package Manager) versions:
1 2 |
node -v npm -v |
Step 3: Install Build Tools
To compile and install native add-ons from npm, you’ll need to install the build-essential package:
1 |
sudo apt install -y build-essential |
Installing Ionic
Step 1: Install Ionic CLI
Ionic’s Command Line Interface (CLI) is essential for creating and managing Ionic projects. Install it globally using npm:
1 |
sudo npm install -g @ionic/cli |
Verify the installation by checking the Ionic version:
1 |
ionic -v |
Step 2: Create a New Ionic Project
Now, let's create a new Ionic project. Open your terminal and run the following command:
1 |
ionic start myApp tabs |
This command initializes a new Ionic project named "myApp" using the "tabs" template. You can choose other templates like "blank" or "sidemenu" based on your project needs.
Navigate to your project directory:
1 |
cd myApp |
Building Your First Ionic App
Step 1: Serve the App
To see your app in action, run:
1 |
ionic serve |
This command starts a local development server and opens your app in a web browser. You should see the default Ionic tabs template.
Step 2: Modify the Home Page
Open the src/app/home/home.page.html
file and make some changes to personalize your app. For example, you can add a welcome message:
1 2 3 4 5 6 7 8 9 10 11 12 |
<ion-header> <ion-toolbar> <ion-title> Home </ion-title> </ion-toolbar> </ion-header> <ion-content class="ion-padding"> <h2>Welcome to myApp!</h2> <p>This is your first Ionic app running on Node.js v16!</p> </ion-content> |
Save the file and your changes should automatically reflect in the browser.
Step 3: Add a New Page
Add a new page to your Ionic app using the CLI:
1 |
ionic generate page about |
This command creates a new page named "about" with the necessary files. Update the src/app/app-routing.module.ts
to include a route for the new page:
1 2 3 4 5 |
const routes: Routes = [ { path: '', redirectTo: 'home', pathMatch: 'full' }, { path: 'home', loadChildren: () => import('./home/home.module').then(m => m.HomePageModule) }, { path: 'about', loadChildren: () => import('./about/about.module').then(m => m.AboutPageModule) }, ]; |
Now, add a link to the new page in the home page:
1 2 3 4 5 |
<ion-content class="ion-padding"> <h2>Welcome to myApp!</h2> <p>This is your first Ionic app running on Node.js v16!</p> <ion-button href="/about">About</ion-button> </ion-content> |
Step 4: Run on a Smartphone
To run your app on a smartphone, you need to add a platform and build the app. For example, to add the Android platform, run:
1 |
ionic capacitor add android |
Build the app:
1 |
ionic build |
Sync the project:
1 |
ionic capacitor sync |
Open the project in Android Studio to run it on a connected device or emulator:
1 |
ionic capacitor open android |
Sample Code: Integrating TypeScript
TypeScript enhances JavaScript by adding static types, which can help catch errors early and improve code quality. Ionic fully supports TypeScript, and integrating it into your project is straightforward.
Example: TypeScript Interface
Create a src/app/interfaces/user.ts
file to define a TypeScript interface:
1 2 3 4 5 |
export interface User { id: number; name: string; email: string; } |
Example: Using the Interface
Modify the src/app/home/home.page.ts
to use the new interface:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 |
import { Component } from '@angular/core'; import { User } from '../interfaces/user'; @Component({ selector: 'app-home', templateUrl: 'home.page.html', styleUrls: ['home.page.scss'], }) export class HomePage { users: User[] = [ { id: 1, name: 'John Doe', email: 'john@example.com' }, { id: 2, name: 'Jane Doe', email: 'jane@example.com' } ]; constructor() {} } |
Update the src/app/home/home.page.html
to display the users:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 |
<ion-header> <ion-toolbar> <ion-title> Home </ion-title> </ion-toolbar> </ion-header> <ion-content class="ion-padding"> <h2>Welcome to myApp!</h2> <p>This is your first Ionic app running on Node.js v16!</p> <ion-list> <ion-item *ngFor="let user of users"> {{ user.name }} ({{ user.email }}) </ion-item> </ion-list> <ion-button href="/about">About</ion-button> </ion-content> |
Advanced: Integrating with Backend Services
Example: Fetch Data from an API
To make your app more dynamic, you might want to fetch data from an external API. For this example, we'll use a simple REST API.
First, install the HttpClient
module:
1 |
npm install @angular/common@latest |
Then, import it in src/app/app.module.ts
:
1 2 3 4 5 6 7 8 9 10 |
import { HttpClientModule } from '@angular/common/http'; @NgModule({ declarations: [AppComponent], entryComponents: [], imports: [BrowserModule, IonicModule.forRoot(), AppRoutingModule, HttpClientModule], providers: [{ provide: RouteReuseStrategy, useClass: IonicRouteStrategy }], bootstrap: [AppComponent], }) export class AppModule {} |
Create a new service src/app/services/user.service.ts
:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 |
import { Injectable } from '@angular/core'; import { HttpClient } from '@angular/common/http'; import { Observable } from 'rxjs'; import { User } from '../interfaces/user'; @Injectable({ providedIn: 'root' }) export class UserService { private apiUrl = 'https://jsonplaceholder.typicode.com/users'; constructor(private http: HttpClient) {} getUsers(): Observable<User[]> { return this.http.get<User[]>(this.apiUrl); } } |
Use the service in src/app/home/home.page.ts
:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 |
import { Component, OnInit } from '@angular/core'; import { UserService } from '../services/user.service'; import { User } from '../interfaces/user'; @Component({ selector: 'app-home', templateUrl: 'home.page.html', styleUrls: ['home.page.scss'], }) export class HomePage implements OnInit { users: User[] = []; constructor(private userService: UserService) {} ngOnInit() { this.userService.getUsers().subscribe((data: User[]) => { this.users = data; }); } } |
Conclusion
By following this guide, you've set up Node.js v16 and Ionic on your Ubuntu machine, created a basic Ionic app, integrated TypeScript, and connected to a backend service. This powerful combination allows you to build robust, cross-platform mobile applications, enhancing your workflow as a digital nomad, programmer, or data scientist.
Keep experimenting with more advanced features and capabilities of Node
.js and Ionic to fully leverage their potential in your projects. Happy coding!