In the decentralized finance (DeFi) ecosystem, sniping is a strategy used by traders to buy newly listed tokens on decentralized exchanges (DEXes) like UniSwap as soon as they become available. This guide will explain UniSwap sniping and provide a Solidity code example to help digital nomads, programmers, and data scientists understand and implement this strategy on the Ethereum network.
Table of Contents
Introduction to UniSwap Sniping
What is Sniping?
Sniping is a trading strategy where bots automatically purchase newly listed tokens immediately after they are listed on a DEX like UniSwap. The goal is to buy the tokens at the lowest possible price before the price rises due to increased demand.
Why Use Sniping?
For traders, sniping can be a lucrative strategy because:
- Early Access: Get tokens at a low initial price.
- Quick Profits: Potentially sell the tokens quickly for a profit as the price rises.
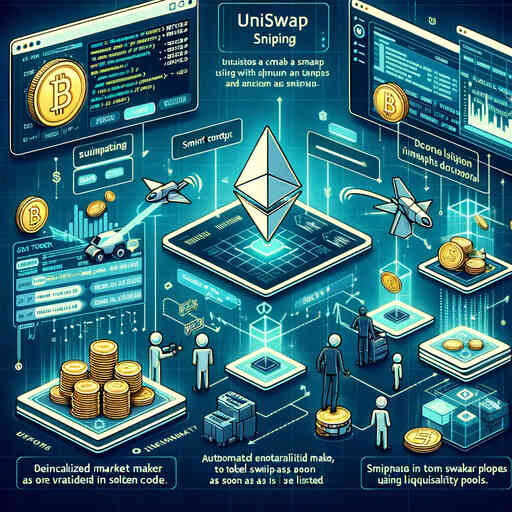
How UniSwap Sniping Works
Automated Execution
Sniping is typically automated using smart contracts or bots that monitor the blockchain for new token listings and execute trades as soon as conditions are met.
Key Components
- Monitoring: Watch for new liquidity pool creations on UniSwap.
- Trading: Execute buy orders immediately when the liquidity pool is created.
- Timing: The success of sniping often depends on executing trades faster than other participants.
Solidity Code for UniSwap Sniping
Prerequisites
Before diving into the code, ensure you have:
- Basic knowledge of Solidity and Ethereum smart contracts.
- A development environment like Remix or Truffle.
- Access to the Ethereum network (e.g., using Infura or Alchemy).
UniSwap Sniping Contract
Below is an example of a UniSwap sniping contract. This contract listens for the creation of new liquidity pools and attempts to buy tokens from these pools immediately.
Sniping Contract
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 |
// SPDX-License-Identifier: MIT pragma solidity ^0.8.0; import "@openzeppelin/contracts/token/ERC20/IERC20.sol"; import "@uniswap/v2-periphery/contracts/interfaces/IUniswapV2Router02.sol"; import "@uniswap/v2-core/contracts/interfaces/IUniswapV2Factory.sol"; contract UniSwapSniper { address public owner; IUniswapV2Router02 public uniswapRouter; IUniswapV2Factory public uniswapFactory; event Sniped(address token, uint256 amountIn, uint256 amountOut); modifier onlyOwner() { require(msg.sender == owner, "Not owner"); _; } constructor(address _uniswapRouter, address _uniswapFactory) { owner = msg.sender; uniswapRouter = IUniswapV2Router02(_uniswapRouter); uniswapFactory = IUniswapV2Factory(_uniswapFactory); } function snipe(address token, uint256 amountIn, uint256 amountOutMin) external onlyOwner { address[] memory path = new address[](2); path[0] = uniswapRouter.WETH(); path[1] = token; IERC20(uniswapRouter.WETH()).approve(address(uniswapRouter), amountIn); uint256[] memory amounts = uniswapRouter.swapExactETHForTokens{ value: amountIn }( amountOutMin, path, address(this), block.timestamp + 300 ); emit Sniped(token, amountIn, amounts[1]); } function withdrawToken(address token) external onlyOwner { uint256 balance = IERC20(token).balanceOf(address(this)); require(balance > 0, "No balance to withdraw"); IERC20(token).transfer(owner, balance); } function withdrawETH() external onlyOwner { payable(owner).transfer(address(this).balance); } receive() external payable {} } |
Explanation
Contract Setup
- Owner: The address that deployed the contract. Only the owner can execute sniping operations.
- UniSwap Router and Factory: Interfaces to interact with UniSwap's router and factory contracts.
Snipe Function
The snipe
function performs the following actions:
- Path Setup: Defines the swap path from WETH to the target token.
- Approval: Approves the UniSwap router to spend the specified amount of WETH.
- Swap Execution: Executes the swap, converting ETH to the target token and ensuring at least
amountOutMin
tokens are received. - Event Emission: Emits a
Sniped
event with details of the transaction.
Withdrawal Functions
These functions allow the owner to withdraw tokens or ETH from the contract.
Deploying the Contract
To deploy this contract, use Remix or Truffle and connect to the Ethereum network (e.g., via Infura).
Using the Sniping Contract
- Deploy the Contract: Deploy the contract using your preferred development environment.
- Provide ETH: Send ETH to the contract to fund sniping transactions.
- Monitor for New Listings: Use off-chain monitoring tools or services to detect new token listings.
- Execute Snipe: Call the
snipe
function with the new token's address and desired amounts.
Risks and Considerations
Risks
- Front-Running: Other traders or bots may execute trades faster, reducing profitability.
- Slippage: Significant price changes between transaction submission and execution.
- Gas Fees: High gas fees during peak times can impact profitability.
Security
Ensure the contract is secure and audited before deploying on the mainnet. Test thoroughly on testnets like Ropsten or Rinkeby.
Conclusion
UniSwap sniping can be a profitable strategy for digital nomads, programmers, and data scientists familiar with Ethereum and Solidity. By understanding and implementing the provided Solidity code, you can create a sniping bot that takes advantage of newly listed tokens on UniSwap. However, always be aware of the associated risks and ensure your strategies are well-tested and secure. Happy trading!