Blockchain technology, and specifically Ethereum, has revolutionized the way we think about decentralized applications and smart contracts. Understanding the intricacies of Ethereum, including gas price, gas limit, and bytecode, is essential for digital nomads, programmers, and data scientists looking to leverage this powerful technology. In this comprehensive guide, we will delve into these concepts, providing practical insights and examples.
Table of Contents
Introduction to Ethereum
What is Ethereum?
Ethereum is a decentralized platform that enables developers to build and deploy smart contracts and decentralized applications (DApps). Unlike Bitcoin, which primarily serves as a digital currency, Ethereum offers a flexible platform for executing complex logic on the blockchain.
Why Ethereum?
For digital nomads, programmers, and data scientists, Ethereum offers a unique opportunity to develop secure, decentralized applications that can run without downtime or third-party interference. The Ethereum network provides a robust ecosystem for innovation and development in the blockchain space.
Key Concepts in Ethereum
Smart Contracts
Smart contracts are self-executing contracts with the terms of the agreement directly written into code. They automatically execute and enforce the terms of the contract when predefined conditions are met.
Gas Price and Gas Limit
To execute transactions and smart contracts on the Ethereum network, you need to pay for computational resources. This is where gas price and gas limit come into play.
- Gas Price: The amount you are willing to pay per unit of gas, typically measured in wei (the smallest unit of ether).
- Gas Limit: The maximum amount of gas you are willing to spend on a transaction or contract execution.
Wei
Wei is the smallest denomination of ether, the cryptocurrency used on the Ethereum network. One ether is equal to 1 quintillion wei (10^18 wei).
Understanding Gas Price and Gas Limit
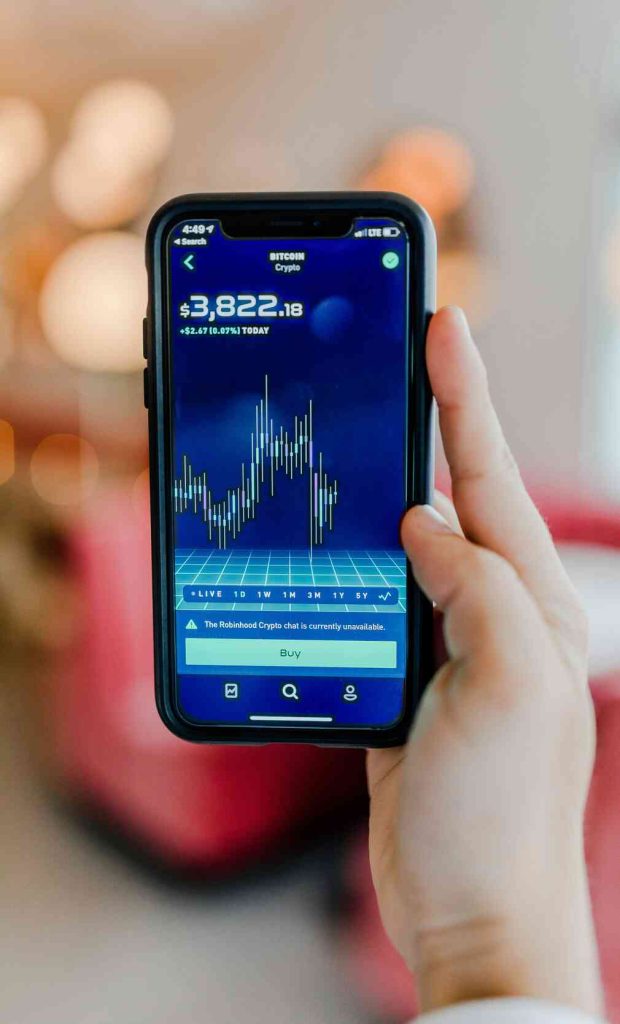
Gas Price
The gas price is set by the user and influences how quickly a transaction is processed by miners. Higher gas prices incentivize miners to prioritize your transaction.
Gas Limit
The gas limit is the maximum amount of gas you are willing to consume for a transaction. This limit ensures you do not spend more gas than necessary, protecting you from excessive costs due to programming errors or unexpected issues.
Calculating Gas Costs
The total cost of a transaction is calculated as:
$$ \text{Total Cost} = \text{Gas Price} \times \text{Gas Used} $$
For example, if the gas price is 20 gwei (20 x 10^9 wei) and the transaction uses 21,000 gas units, the total cost in ether would be:
$$ \text{Total Cost} = 20 \times 10^9 \times 21,000 = 420,000,000,000 \, \text{wei} $$
Converted to ether:
$$ 420,000,000,000 \, \text{wei} = 0.00042 \, \text{ether} $$
Example of Setting Gas Price and Gas Limit
Here is an example of setting the gas price and gas limit for a simple transaction using web3.js, a JavaScript library for interacting with the Ethereum blockchain:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 |
const Web3 = require('web3'); const web3 = new Web3('https://mainnet.infura.io/v3/YOUR_INFURA_PROJECT_ID'); const sendTransaction = async () => { const accounts = await web3.eth.getAccounts(); const receipt = await web3.eth.sendTransaction({ from: accounts[0], to: '0xRecipientAddress', value: web3.utils.toWei('0.01', 'ether'), gasPrice: web3.utils.toWei('20', 'gwei'), gas: 21000, }); console.log('Transaction receipt:', receipt); }; sendTransaction(); |
Smart Contracts and Bytecode
What is Bytecode?
Bytecode is the low-level code that the Ethereum Virtual Machine (EVM) executes. When you write a smart contract in a high-level language like Solidity, it is compiled into bytecode, which is then deployed to the Ethereum blockchain.
Example Smart Contract in Solidity
Here is a simple smart contract written in Solidity:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 |
pragma solidity ^0.8.0; contract SimpleStorage { uint256 private storedData; event DataStored(uint256 data); function set(uint256 x) public { storedData = x; emit DataStored(x); } function get() public view returns (uint256) { return storedData; } } |
Deploying the Smart Contract
To deploy the smart contract, you need to compile it and send the resulting bytecode to the Ethereum network. Here’s how you can do it using web3.js and the Solidity compiler solc:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 |
const Web3 = require('web3'); const solc = require('solc'); const fs = require('fs'); const web3 = new Web3('https://mainnet.infura.io/v3/YOUR_INFURA_PROJECT_ID'); const source = fs.readFileSync('SimpleStorage.sol', 'utf8'); const compiled = solc.compile(source, 1); const abi = JSON.parse(compiled.contracts[':SimpleStorage'].interface); const bytecode = compiled.contracts[':SimpleStorage'].bytecode; const deployContract = async () => { const accounts = await web3.eth.getAccounts(); const simpleStorage = new web3.eth.Contract(abi); const deployedContract = await simpleStorage.deploy({ data: bytecode, }) .send({ from: accounts[0], gas: 1500000, gasPrice: web3.utils.toWei('20', 'gwei'), }); console.log('Contract deployed at address:', deployedContract.options.address); }; deployContract(); |
Interacting with the Smart Contract
Once deployed, you can interact with the smart contract by calling its methods. Here’s an example of how to set and get data in the SimpleStorage contract:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 |
const Web3 = require('web3'); const web3 = new Web3('https://mainnet.infura.io/v3/YOUR_INFURA_PROJECT_ID'); const contractABI = [/* ABI array here */]; const contractAddress = 'YOUR_CONTRACT_ADDRESS'; const simpleStorage = new web3.eth.Contract(contractABI, contractAddress); const interactWithContract = async () => { const accounts = await web3.eth.getAccounts(); // Set data await simpleStorage.methods.set(42).send({ from: accounts[0], gas: 150000, gasPrice: web3.utils.toWei('20', 'gwei'), }); // Get data const data = await simpleStorage.methods.get().call(); console.log('Stored data:', data); }; interactWithContract(); |
Gas Price and Gas Limit in Smart Contracts
Importance of Gas Management
Managing gas price and gas limit is crucial when deploying and interacting with smart contracts. Incorrect settings can lead to failed transactions or excessive costs. Here are some tips for efficient gas management:
- Estimate Gas Usage: Use web3.js to estimate the gas required for a transaction.
- Adjust Gas Price: Monitor network congestion and adjust the gas price accordingly to ensure timely processing.
Example: Estimating Gas
You can estimate the gas required for a transaction using the estimateGas
method:
1 2 3 4 5 6 7 8 9 10 11 |
const estimateGas = async () => { const accounts = await web3.eth.getAccounts(); const gasEstimate = await simpleStorage.methods.set(42).estimateGas({ from: accounts[0], }); console.log('Estimated Gas:', gasEstimate); }; estimateGas(); |
Example: Dynamic Gas Price
You can dynamically set the gas price based on network conditions:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 |
const setDynamicGasPrice = async () => { const gasPrice = await web3.eth.getGasPrice(); console.log('Current Gas Price:', gasPrice); const accounts = await web3.eth.getAccounts(); await simpleStorage.methods.set(42).send({ from: accounts[0], gas: 150000, gasPrice: gasPrice, // Set dynamically }); }; setDynamicGasPrice(); |
Managing Gas Costs Efficiently
Understanding and managing gas costs is crucial for optimizing the performance and cost-efficiency of smart contracts and transactions. This is particularly important for digital nomads who may have limited resources and need to maximize their returns on investment.
Conclusion
Ethereum’s capabilities, including its smart contracts, gas price, gas limit, and bytecode execution, offer immense potential for digital nomads, programmers, and data scientists. By understanding these concepts and leveraging the practical examples provided, you can harness the power of Ethereum to develop innovative applications, manage transactions efficiently, and explore new frontiers in blockchain technology. Whether you’re building DApps or optimizing data integrity, Ethereum provides the tools and framework to achieve your goals. Happy coding!