In the world of blockchain and decentralized applications, Solidity and Web3.js have become essential tools for developers. This guide is tailored for digital nomads, programmers, and data scientists who want to dive into Solidity with Web3.js for JavaScript development. We’ll explore how to set up your environment, write smart contracts in Solidity, and interact with them using Web3.js.
Table of Contents
Introduction to Solidity and Web3.js
What is Solidity?
Solidity is a statically-typed programming language designed for developing smart contracts that run on the Ethereum Virtual Machine (EVM). It’s the language of choice for writing decentralized applications (DApps) on the Ethereum blockchain.
What is Web3.js?
Web3.js is a collection of libraries that allows you to interact with a local or remote Ethereum node using HTTP, IPC, or WebSocket. It’s essential for developers who want to interact with Ethereum smart contracts from a JavaScript environment.
Why Learn Solidity with Web3.js?
For digital nomads, programmers, and data scientists, mastering Solidity with Web3.js opens up numerous opportunities in the blockchain space. Whether you’re developing DApps, exploring decentralized finance (DeFi), or working on blockchain analytics, these skills are invaluable.
Setting Up Your Development Environment
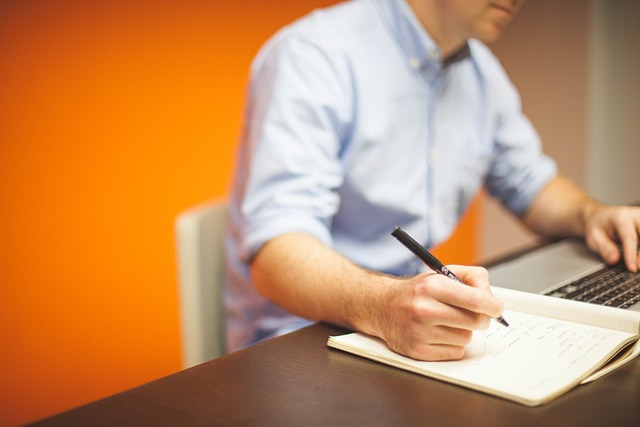
Prerequisites
Before diving in, ensure you have the following:
- Node.js and NPM: These are essential for managing your development environment.
- Truffle: A development framework for Ethereum.
- Ganache: A personal blockchain for Ethereum development.
Installing Node.js and NPM
Install Node.js and NPM (Node Package Manager) from the official website or use a package manager.
1 2 |
sudo apt update sudo apt install nodejs npm |
Verify the installation:
1 2 |
node -v npm -v |
Installing Truffle and Ganache
Install Truffle globally using NPM:
1 |
npm install -g truffle |
Install Ganache:
1 |
npm install -g ganache-cli |
Setting Up a New Truffle Project
Create a new directory for your project and initialize a Truffle project:
1 2 3 |
mkdir myDapp cd myDapp truffle init |
Installing Web3.js
Install Web3.js in your project:
1 |
npm install web3 |
Writing Your First Smart Contract in Solidity
Creating the Smart Contract
Create a new file SimpleStorage.sol
in the contracts
directory of your Truffle project:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 |
pragma solidity ^0.8.0; contract SimpleStorage { uint256 private storedData; event DataStored(uint256 data); function set(uint256 x) public { storedData = x; emit DataStored(x); } function get() public view returns (uint256) { return storedData; } } |
Compiling the Contract
Compile the smart contract using Truffle:
1 |
truffle compile |
Deploying the Contract
Create a new migration file in the migrations
directory:
1 2 3 4 5 |
const SimpleStorage = artifacts.require("SimpleStorage"); module.exports = function(deployer) { deployer.deploy(SimpleStorage); }; |
Deploy the contract to Ganache:
1 |
truffle migrate --network development |
Interacting with the Smart Contract Using Web3.js
Connecting to Ethereum Network
Create a new JavaScript file interact.js
in your project root:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 |
const Web3 = require('web3'); const web3 = new Web3('http://localhost:7545'); // Ganache default port const contractABI = [/* ABI array here */]; const contractAddress = 'YOUR_CONTRACT_ADDRESS'; const simpleStorage = new web3.eth.Contract(contractABI, contractAddress); const interactWithContract = async () => { const accounts = await web3.eth.getAccounts(); // Set data await simpleStorage.methods.set(42).send({ from: accounts[0], gas: 150000, }); // Get data const data = await simpleStorage.methods.get().call(); console.log('Stored data:', data); }; interactWithContract(); |
Replace /* ABI array here */
with the ABI of your contract and YOUR_CONTRACT_ADDRESS
with the deployed contract address from Ganache.
Running the Script
Run the interact.js
script:
1 |
node interact.js |
Expected Output
1 |
Stored data: 42 |
Practical Applications
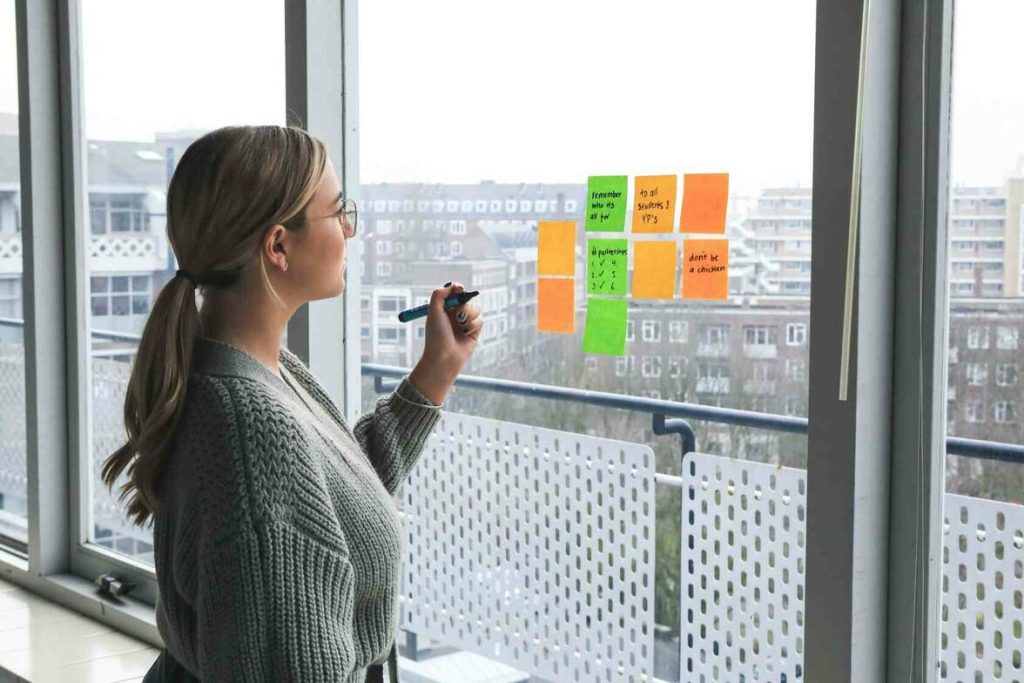
Decentralized Applications (DApps)
For digital nomads, developing DApps allows you to create applications that are not controlled by a single entity, offering transparency and security. With Solidity and Web3.js, you can build applications ranging from simple voting systems to complex decentralized exchanges.
Blockchain Analytics
Data scientists can use Web3.js to analyze blockchain data, extracting insights and trends from transaction histories, smart contract interactions, and more. This capability is crucial for blockchain research and development.
Smart Contract Development
Programmers can leverage Solidity to develop robust smart contracts that automate processes and enforce agreements without intermediaries. This includes financial contracts, supply chain tracking, and more.
Advanced Topics
Event Handling
Smart contracts can emit events that are logged on the blockchain. You can listen for these events using Web3.js.
Solidity Code
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 |
pragma solidity ^0.8.0; contract SimpleStorage { uint256 private storedData; event DataStored(uint256 data); function set(uint256 x) public { storedData = x; emit DataStored(x); } function get() public view returns (uint256) { return storedData; } } |
Web3.js Code
1 2 3 4 5 6 7 8 |
simpleStorage.events.DataStored({ fromBlock: 0 }, function (error, event) { console.log(event); }) .on('data', function (event) { console.log('Event data:', event.returnValues); }); |
Handling Transactions
Managing transactions efficiently is crucial for Ethereum development. Here’s how to estimate gas and handle transactions.
Estimating Gas
1 2 3 4 5 6 7 8 9 10 11 |
const estimateGas = async () => { const accounts = await web3.eth.getAccounts(); const gasEstimate = await simpleStorage.methods.set(42).estimateGas({ from: accounts[0], }); console.log('Estimated Gas:', gasEstimate); }; estimateGas(); |
Sending Transactions
1 2 3 4 5 6 7 8 9 10 11 12 |
const sendTransaction = async () => { const accounts = await web3.eth.getAccounts(); const receipt = await simpleStorage.methods.set(42).send({ from: accounts[0], gas: 150000, }); console.log('Transaction receipt:', receipt); }; sendTransaction(); |
Conclusion
Mastering Solidity with Web3.js is a valuable skill set for digital nomads, programmers, and data scientists. This guide has covered the basics of setting up your development environment, writing and deploying smart contracts, and interacting with them using Web3.js. By leveraging these tools, you can develop decentralized applications, analyze blockchain data, and automate processes with smart contracts.
Continue exploring and experimenting with Solidity and Web3.js to unlock the full potential of blockchain technology. Happy coding!