In the digital age, converting images to text using Optical Character Recognition (OCR) technology has become an essential skill. Whether you're a programmer, a data scientist, or simply someone looking to digitize documents, OCR can save you time and effort. This article will guide you through setting up OCR on Ubuntu, focusing on multilingual capabilities to handle texts in various languages.
Table of Contents
Introduction to OCR
OCR stands for Optical Character Recognition. It's a technology that converts different types of documents, such as scanned paper documents, PDF files, or images captured by a digital camera, into editable and searchable data. OCR is particularly useful for digitizing printed documents so that they can be electronically edited, searched, and stored more compactly.
Why Use OCR on Ubuntu?
Ubuntu, a popular Linux distribution, provides a stable and powerful environment for running OCR software. The open-source nature of Ubuntu makes it an excellent choice for those looking to implement OCR solutions without incurring high costs. Additionally, the flexibility and customizability of Ubuntu allow for fine-tuning the OCR process to suit specific needs, including multilingual text recognition.
Setting Up OCR on Ubuntu
Installing Tesseract OCR
Tesseract is one of the most popular open-source OCR engines. It's known for its accuracy and support for multiple languages. To install Tesseract on Ubuntu, use the following commands:
1 2 3 |
sudo apt update sudo apt install tesseract-ocr sudo apt install tesseract-ocr-all |
The tesseract-ocr-all
package includes support for a wide range of languages, making it ideal for multilingual OCR tasks.
Installing Python and Pytesseract
For those who prefer scripting their OCR tasks, Python combined with the Pytesseract library offers a powerful solution. Pytesseract acts as a wrapper for Tesseract, allowing you to use its capabilities directly from Python scripts.
1 2 3 |
sudo apt install python3-pip pip3 install pytesseract pip3 install Pillow |
Performing OCR on Images
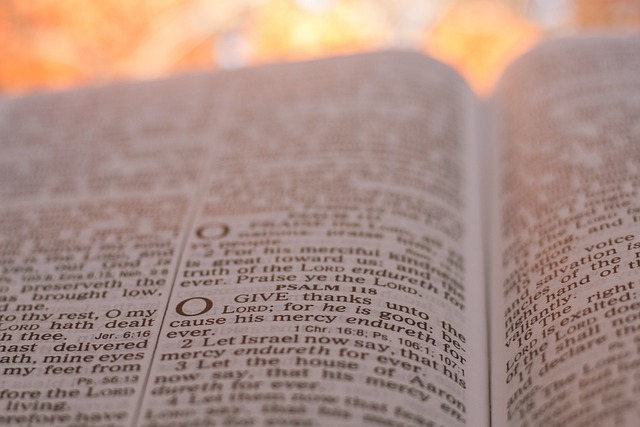
Basic OCR with Tesseract
Once Tesseract is installed, you can start using it to extract text from images. The basic syntax for running Tesseract from the command line is:
1 |
tesseract image.png output |
This command will process image.png
and save the recognized text in a file named output.txt
.
OCR with Python and Pytesseract
To perform OCR using Python, you can create a script that utilizes Pytesseract. Here's a simple example:
1 2 3 4 5 6 7 8 9 10 11 |
from PIL import Image import pytesseract # Open an image file image = Image.open('image.png') # Use Tesseract to do OCR on the image text = pytesseract.image_to_string(image) # Print the extracted text print(text) |
This script opens an image file, processes it with Tesseract, and prints the extracted text.
Handling Multilingual Texts
Specifying Languages with Tesseract
Tesseract can handle multiple languages, but you need to specify which languages to use. This is done using the -l
parameter followed by the language codes. For example, to recognize English and Spanish text in the same image:
1 |
tesseract image.png output -l eng+spa |
Multilingual OCR with Pytesseract
In Python, you can specify languages by passing the lang
parameter to the image_to_string
function. Here's an example:
1 2 3 4 5 6 7 8 9 10 11 |
from PIL import Image import pytesseract # Open an image file image = Image.open('multilingual_image.png') # Use Tesseract to do OCR on the image with multiple languages text = pytesseract.image_to_string(image, lang='eng+spa') # Print the extracted text print(text) |
This script tells Tesseract to use both English and Spanish for text recognition.
Enhancing OCR Accuracy
Preprocessing Images
OCR accuracy can be significantly improved by preprocessing images. This includes tasks such as resizing, converting to grayscale, and thresholding. Using the Python Imaging Library (PIL), you can preprocess images before running OCR.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 |
from PIL import Image, ImageEnhance, ImageFilter import pytesseract # Open an image file image = Image.open('image.png') # Convert the image to grayscale image = image.convert('L') # Enhance the image image = image.filter(ImageFilter.SHARPEN) # Use Tesseract to do OCR on the preprocessed image text = pytesseract.image_to_string(image) # Print the extracted text print(text) |
Using Page Segmentation Modes
Tesseract offers different page segmentation modes (PSMs) to optimize text recognition based on the layout of the text. For example, PSM 6 treats the image as a single block of text, while PSM 3 fully automatically segments the page. You can specify the PSM with the --psm
parameter.
1 |
tesseract image.png output --psm 6 |
In Python, you can set the PSM using the config
parameter:
1 2 3 4 5 6 7 8 9 10 11 12 |
from PIL import Image import pytesseract # Open an image file image = Image.open('image.png') # Use Tesseract to do OCR with a specific PSM custom_config = r'--psm 6' text = pytesseract.image_to_string(image, config=custom_config) # Print the extracted text print(text) |
Advanced Multilingual OCR
Detecting Languages Automatically
Tesseract has a feature that allows it to detect the language automatically, although it might not be as accurate as specifying the languages explicitly. To enable automatic language detection, use the -l osd
option.
1 |
tesseract image.png output -l osd |
In Python:
1 2 3 4 5 6 7 8 9 10 11 |
from PIL import Image import pytesseract # Open an image file image = Image.open('image.png') # Use Tesseract to do OCR with automatic language detection text = pytesseract.image_to_string(image, lang='osd') # Print the extracted text print(text) |
Combining Languages with Custom Dictionaries
For better accuracy with specialized vocabularies or technical terms, you can use custom dictionaries with Tesseract. This involves creating a plain text file with your custom words and configuring Tesseract to use it during OCR.
1 |
tesseract image.png output --user-words custom_words.txt -l eng+spa |
Practical Applications of OCR
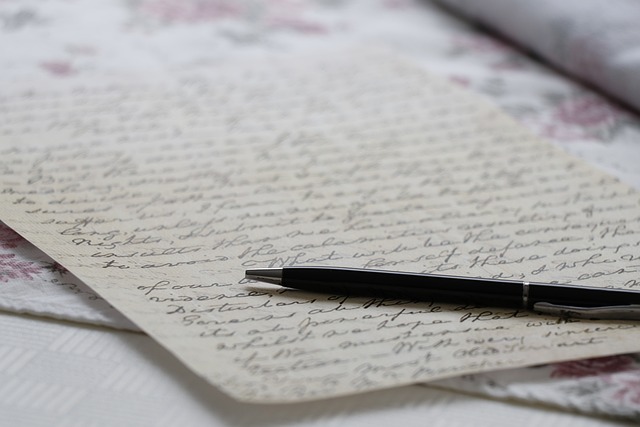
Digitizing Printed Documents
OCR can be used to digitize printed documents, making them searchable and editable. This is particularly useful for archiving old books, legal documents, and academic papers.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 |
from PIL import Image import pytesseract # Open an image file image = Image.open('document.png') # Convert the image to grayscale image = image.convert('L') # Enhance the image image = image.filter(ImageFilter.SHARPEN) # Use Tesseract to do OCR on the preprocessed image text = pytesseract.image_to_string(image) # Print the extracted text print(text) |
Translating Text
Once text is extracted using OCR, it can be translated using various translation libraries in Python, such as Google Translate API.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 |
from googletrans import Translator from PIL import Image import pytesseract # Open an image file image = Image.open('multilingual_image.png') # Extract text from image text = pytesseract.image_to_string(image, lang='eng+spa') # Initialize the translator translator = Translator() # Translate the text translated_text = translator.translate(text, dest='fr') # Print the translated text print(translated_text.text) |
Analyzing Historical Documents
OCR can be used to digitize and analyze historical documents. This makes it easier to search through archives and perform textual analysis on old manuscripts.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 |
from PIL import Image import pytesseract # Open an image file image = Image.open('historical_document.png') # Convert the image to grayscale image = image.convert('L') # Enhance the image image = image.filter(ImageFilter.SHARPEN) # Use Tesseract to do OCR on the preprocessed image text = pytesseract.image_to_string(image, lang='eng') # Print the extracted text print(text) |
Conclusion
OCR technology is a powerful tool for converting images to text, and with the right setup, Ubuntu provides an excellent environment for performing OCR tasks. By using Tesseract and Pytesseract, you can easily handle multilingual texts and improve OCR accuracy with preprocessing techniques. Whether you’re digitizing documents, translating text, or analyzing historical manuscripts, OCR on Ubuntu offers a versatile and efficient solution.