Solidity, the programming language for writing smart contracts on the Ethereum blockchain, offers powerful features for managing state and variables. To write efficient and secure smart contracts, it's crucial to understand the differences between memory and storage, how to handle variables, and how to use assertions and tests effectively. This article dives deep into these concepts with practical examples, providing a comprehensive guide for developers.
Table of Contents
Introduction to Solidity
Solidity is a statically-typed programming language designed for developing smart contracts that run on the Ethereum Virtual Machine (EVM). It supports complex user-defined types, libraries, and inheritance, making it a robust choice for blockchain development.
Memory and Storage in Solidity
Memory
Memory in Solidity is a temporary place where data is stored during function execution. It is erased between (external) function calls and is not persistent. Variables stored in memory are cheaper to access and modify but do not retain their values outside the function scope.
Storage
Storage, on the other hand, is a persistent place where data is stored on the blockchain. Variables stored in storage remain until they are explicitly changed or deleted. Accessing and modifying storage variables is more expensive compared to memory due to the gas cost associated with writing data to the blockchain.
Example of Memory and Storage
Consider the following example that demonstrates the use of memory and storage:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 |
pragma solidity ^0.8.0; contract MemoryStorageExample { uint[] public storageArray; function addToStorageArray(uint value) public { storageArray.push(value); } function modifyInMemory() public view returns(uint[] memory) { uint[] memory tempArray = storageArray; tempArray[0] = 999; return tempArray; } function modifyInStorage() public { storageArray[0] = 999; } } |
In this example, addToStorageArray
modifies the storageArray
directly, which is stored in storage. The modifyInMemory
function creates a temporary copy of storageArray
in memory, modifies it, and returns it. The original storageArray
remains unchanged. The modifyInStorage
function, however, directly modifies storageArray
in storage.
Variable Types in Solidity
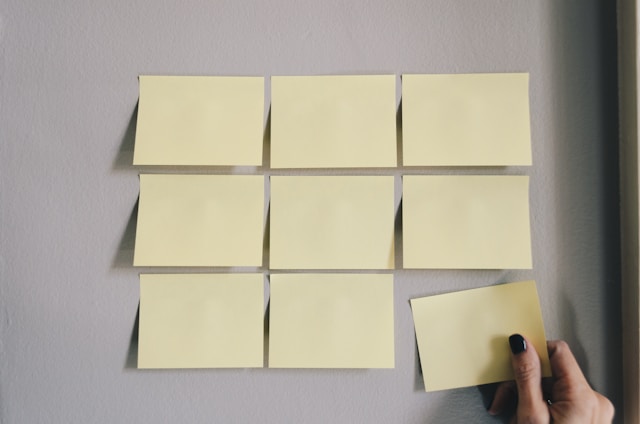
State Variables
State variables are permanently stored in contract storage. They are declared outside of functions and persist for the lifetime of the contract.
1 2 3 4 5 6 7 8 9 |
pragma solidity ^0.8.0; contract StateVariableExample { uint public stateVariable; function setStateVariable(uint value) public { stateVariable = value; } } |
Local Variables
Local variables are temporary and only exist within the function they are declared. They are stored in memory and are cheaper to use than state variables.
1 2 3 4 5 6 7 8 |
pragma solidity ^0.8.0; contract LocalVariableExample { function calculateSum(uint a, uint b) public pure returns (uint) { uint sum = a + b; return sum; } } |
Global Variables
Global variables provide information about the blockchain and are available throughout the contract. Examples include msg.sender
(the address of the caller) and block.timestamp
(the current block timestamp).
1 2 3 4 5 6 7 |
pragma solidity ^0.8.0; contract GlobalVariableExample { function getBlockTimestamp() public view returns (uint) { return block.timestamp; } } |
Using Assert for Testing in Solidity
Assertions are used to test assumptions made in the code. The assert
function is used to ensure that conditions hold true. If the condition fails, the transaction is reverted, and all changes made are undone.
Example of Using Assert
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 |
pragma solidity ^0.8.0; contract AssertExample { uint public value; function setValue(uint _value) public { value = _value; assert(value == _value); } function testAssert() public { uint a = 2; uint b = 3; uint result = a + b; assert(result == 5); } } |
In this example, assert
is used to check that value
is correctly set and that the result of the addition operation is as expected.
Writing Tests in Solidity
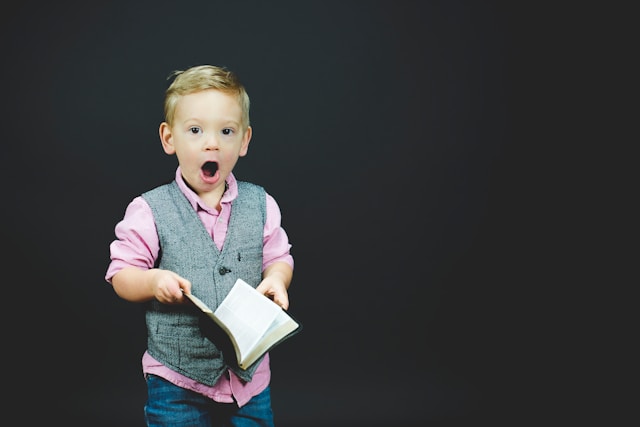
Testing smart contracts is crucial to ensure they behave as expected. Solidity supports unit testing using testing frameworks such as Truffle or Hardhat. Here, we’ll see an example using Truffle to test the MemoryStorageExample
contract.
Example of Writing Tests
First, install Truffle and set up a project:
1 2 |
npm install -g truffle truffle init |
Create a new test file test/memoryStorageExampleTest.js
:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 |
const MemoryStorageExample = artifacts.require("MemoryStorageExample"); contract("MemoryStorageExample", (accounts) => { it("should add value to storage array", async () => { const instance = await MemoryStorageExample.deployed(); await instance.addToStorageArray(42); const result = await instance.storageArray(0); assert.equal(result.toNumber(), 42, "The value 42 was not stored."); }); it("should modify value in memory", async () => { const instance = await MemoryStorageExample.deployed(); const result = await instance.modifyInMemory(); assert.equal(result[0].toNumber(), 999, "The value was not modified in memory."); }); it("should modify value in storage", async () => { const instance = await MemoryStorageExample.deployed(); await instance.modifyInStorage(); const result = await instance.storageArray(0); assert.equal(result.toNumber(), 999, "The value was not modified in storage."); }); }); |
Run the tests using Truffle:
1 |
truffle test |
This will execute the tests and provide feedback on whether the contract behaves as expected.
Best Practices for Using Memory and Storage
Understanding when to use memory versus storage is crucial for optimizing gas costs and ensuring efficient contract execution. Here are some best practices:
- Use memory for temporary variables and computations that do not need to persist beyond the function call.
- Use storage for variables that need to persist across function calls and transactions.
- Minimize the use of storage variables to reduce gas costs, as writing to storage is significantly more expensive than using memory.
- Consider the scope and lifetime of your data when deciding between memory and storage.
Conclusion
Mastering Solidity involves understanding the intricacies of memory, storage, and variables. By leveraging these concepts, you can write efficient and secure smart contracts. Utilizing assertions and tests ensures that your contracts behave as expected, providing confidence in their functionality. Whether you're a seasoned developer or just starting with Solidity, these tools and techniques will help you create robust smart contracts on the Ethereum blockchain.