For digital nomads, programmers, and data scientists, utilizing the power of the Twitter (X) API can open up a world of possibilities, from searching users to trend tracking. This guide will explore the Twitter (X) API, demonstrate how to use it with Python, and discuss its limitations and practical applications.
Table of Contents
Introduction to the Twitter (X) API
What is the Twitter (X) API?
The Twitter (X) API allows developers to interact with Twitter data programmatically. You can use the API to access tweets, user profiles, and other Twitter data in real-time. This is particularly useful for creating applications that analyze trends, or gather data for research purposes.
Why Use the Twitter (X) API?
For digital nomads, programmers, and data scientists, the Twitter (X) API provides a powerful tool for data collection and analysis. Whether you are building a machine learning model, conducting social research, or developing a new app, the Twitter (X) API can help you gather the data you need.
Setting Up the Twitter (X) API
Prerequisites
Before you start, ensure you have the following:
- A Twitter developer account
- Python installed on your system
- Basic knowledge of Python programming
Creating a Twitter Developer Account
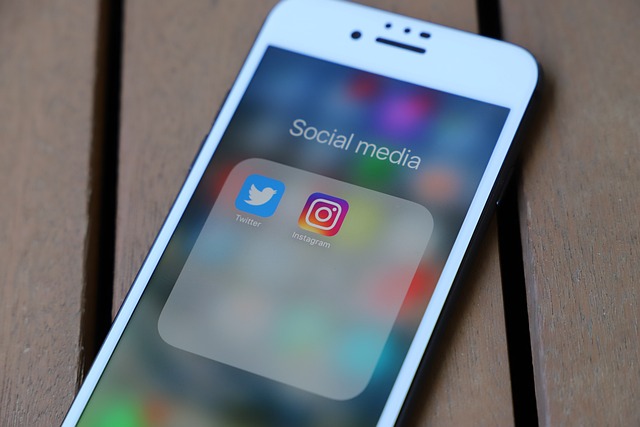
To use the Twitter (X) API, you need to create a developer account and set up a project. Follow these steps:
- Go to the Twitter Developer website and sign up for a developer account.
- Create a new project and app.
- Generate the API keys and tokens: API key, API secret key, Access token, and Access token secret.
Installing Required Libraries
For this tutorial, we will use the tweepy
library to interact with the Twitter (X) API. Install it using pip:
1 |
pip install tweepy |
Using the Twitter (X) API with Python
Authenticating with the API
First, authenticate with the Twitter (X) API using your API keys and tokens. Create a Python script and add the following code:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 |
import tweepy # Replace these values with your own API keys and tokens api_key = 'your_api_key' api_secret_key = 'your_api_secret_key' access_token = 'your_access_token' access_token_secret = 'your_access_token_secret' # Authenticate to the Twitter API auth = tweepy.OAuth1UserHandler(api_key, api_secret_key, access_token, access_token_secret) api = tweepy.API(auth) # Verify authentication try: api.verify_credentials() print("Authentication OK") except: print("Error during authentication") |
Fetching Tweets
Now that you're authenticated, you can fetch tweets. Here's an example of how to fetch recent tweets containing a specific keyword:
1 2 3 4 5 6 7 8 9 10 |
import tweepy # Function to fetch tweets def fetch_tweets(keyword, count): tweets = api.search_tweets(q=keyword, count=count) for tweet in tweets: print(f"{tweet.user.name}:{tweet.text}") # Fetch tweets containing the keyword 'Python' fetch_tweets('Python', 10) |
Fetching User Profile Information
You can also fetch user profile information. Here's an example:
1 2 3 4 5 6 7 8 9 10 11 12 |
import tweepy # Function to fetch user profile def fetch_user_profile(username): user = api.get_user(screen_name=username) print(f"User: {user.name}") print(f"Bio: {user.description}") print(f"Location: {user.location}") print(f"Followers: {user.followers_count}") # Fetch user profile for 'TwitterDev' fetch_user_profile('TwitterDev') |
Streaming Tweets in Real-Time
For real-time data, you can use the streaming API. Here's an example of how to stream tweets containing a specific keyword:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 |
import tweepy # Stream Listener class class MyStreamListener(tweepy.StreamListener): def on_status(self, status): print(f"{status.user.name}:{status.text}") def on_error(self, status_code): if status_code == 420: return False # Function to stream tweets def stream_tweets(keyword): myStreamListener = MyStreamListener() myStream = tweepy.Stream(auth=api.auth, listener=myStreamListener) myStream.filter(track=[keyword]) # Stream tweets containing the keyword 'Python' stream_tweets('Python') |
Understanding API Limits
Rate Limits
The Twitter (X) API has rate limits to prevent abuse and ensure fair usage. These limits specify how many requests you can make within a specific time period.
Here is a table summarizing some of the key rate limits for the standard Twitter API:
API Endpoint | Rate Limit |
---|---|
Search Tweets | 450 requests per 15 minutes |
User Timeline | 900 requests per 15 minutes |
Home Timeline | 15 requests per 15 minutes |
Get Followers List | 15 requests per 15 minutes |
Get Friends List | 15 requests per 15 minutes |
Post a Tweet | 300 tweets per 3 hours |
Retweets/likes | 1000 tweets per 24 hours |
Direct Messages (send) | 15 requests per 15 minutes |
Streaming API Connection | 1 connection per account |
Handling Rate Limits
To handle rate limits, you can check the remaining number of requests and the reset time:
1 2 3 4 |
rate_limit_status = api.rate_limit_status() search_limits = rate_limit_status['resources']['search']['/search/tweets'] print(f"Remaining: {search_limits['remaining']}") print(f"Reset Time: {search_limits['reset']}") |
Implementing a wait mechanism to avoid hitting the rate limit:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 |
import time def fetch_tweets(keyword, count): while True: tweets = api.search_tweets(q=keyword, count=count) for tweet in tweets: print(f"{tweet.user.name}:{tweet.text}") rate_limit_status = api.rate_limit_status() search_limits = rate_limit_status['resources']['search']['/search/tweets'] if search_limits['remaining'] == 0: reset_time = search_limits['reset'] - time.time() print(f"Rate limit reached. Waiting for {reset_time} seconds.") time.sleep(reset_time) else: break # Fetch tweets containing the keyword 'Python' fetch_tweets('Python', 10) |
Practical Applications
Trend Analysis
You can track trending topics on Twitter using the trends_place
method:
1 2 3 4 5 6 7 8 |
# Function to fetch trending topics def fetch_trending_topics(location_id): trends = api.trends_place(location_id) for trend in trends[0]['trends']: print(trend['name']) # Fetch trending topics for a specific location (e.g., Worldwide: 1) fetch_trending_topics(1) |
Data Collection for Research
Data scientists can collect large datasets for research purposes. Store the fetched tweets in a database for further analysis:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 |
import sqlite3 # Create a SQLite database conn = sqlite3.connect('tweets.db') c = conn.cursor() c.execute('''CREATE TABLE IF NOT EXISTS tweets (username TEXT, tweet TEXT)''') conn.commit() # Function to fetch and store tweets def fetch_and_store_tweets(keyword, count): tweets = api.search_tweets(q=keyword, count=count) for tweet in tweets: c.execute("INSERT INTO tweets (username, tweet) VALUES (?, ?)", (tweet.user.name, tweet.text)) conn.commit() # Fetch and store tweets containing the keyword 'Python' fetch_and_store_tweets('Python', 10) # Close the database connection conn.close() |
Searching Users by Keywords
Another practical example is searching for users by keywords and listing the results. This can be useful for finding influencers, potential collaborators, or monitoring competitors.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 |
import tweepy # Function to search users by keyword def search_users(keyword, count): users = api.search_users(q=keyword, count=count) for user in users: print(f"Username: {user.screen_name}") print(f"Name: {user.name}") print(f"Bio: {user.description}") print(f"Location: {user.location}") print(f"Followers: {user.followers_count}") print("-----") # Search for users related to 'Data Science' search_users('Data Science',10) |
Conclusion
The Twitter (X) API provides a powerful way for digital nomads, programmers, and data scientists to access and analyze real-time data from Twitter. By using Python and libraries like tweepy
, you can fetch tweets, analyze sentiments, track trends, search for users, and gather data for research. This guide has covered the basics of setting up and using the Twitter (X) API, handling rate limits, and practical applications of the data. Keep exploring and experimenting to unlock the full potential of Twitter data in your projects.